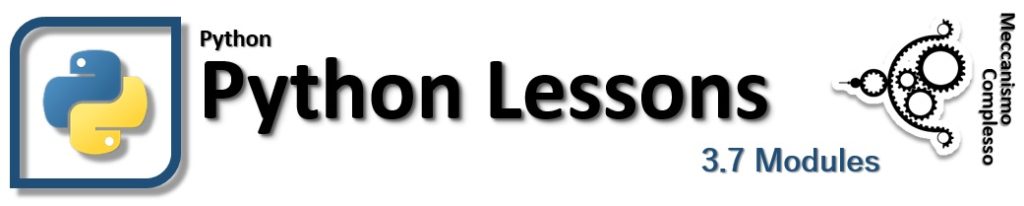
Modules
Modules are pieces of code written by other programmers that perform functions that can be useful in many cases, therefore reusable in other codes. Each of these modules generally performs a series of specialized operations in a given field that can be useful to reuse without having to rewrite the code all over again.
Indeed, sometimes these modules are so complex that they provide a good way to avoid getting lost in the resolution of complicated algorithms that have already been implemented by others.
Each module then provides a series of functions that require the import of the module to be used. This can be done thanks to the import clause.
import modulename
For example there is a module that allows you to manage random numbers called random. This module provides a range of tools including the generation of random numbers. If in creating our code we need to generate random numbers we can simply import the random module and then use its randint () function.
For example, you can generate 10 random numbers ranging from 1 to 100
import random
for i in range(10):
print(random.randint(1,100))
Eseguiamo
>>>
16
53
63
65
28
84
61
58
37
77
You have simply obtained 10 random numbers in a very simple way.
Import a single function from a form
Sometimes you need only a certain function of a module. It is therefore possible to import it from a module using the from clause.
from modulo import nomefunzione
And then make the function call in this way
modulo.nomefunzione
In the case of the random number generator we could write like this:
from random import randint
for i in range(10):
print(randint(1,100))
As you can see, in this case, there is no longer any need to specify the module name during the function call.
Furthermore it is possible to import multiple functions (or objects) from the same module, just put them in sequence separated by a comma.
from modulo import nomefunzione1, nomefunzione2, nomefunzione3
Import all objects from a module.
Often you will be able to see the following case
1) from modulo import *
with this command (no error) all the functions and objects belonging to a module are imported. But what is the difference with respect to the following command?
2) import modulo
Well the first case the function calls do not require the name of the module from which they derive. Unlike the second case in which, instead, the function calls explicitly require referencing to the appearance module
1) nomefunzione()
2) nomemodulo.nomefunzione()
But the first case, that is case 1 (even if it works), is not correct because it could happen that different modules have functions with the same name, and then the last module imported will overwrite objects and functions with the same name of the modules previous, creating havoc and confusion during the code execution.
It is therefore recommended to always follow method 2 if you want to import a module in your interest.
Import a module using an alias
You have just seen that to use a function call of a module it is necessary to refer it to the module itself. Sometimes, however, some modules have a really long name and bringing it back numerous times in the code can be really tedious. An easy way to solve this problem is to import a module using an alias of a few characters, and to do so use the as clause. From then on, calls to the functions will be referenced to the alias instead of the original module name.
So instead of writing the call to the function as follows
thisisaverylongmodulename.methodname()
you can solve it by writing this way
import thisisaverylongmodulename as qm
qm.methodname()
⇐ Go to Python Lesson 3.6 – Functions as objects
Go to Python Lesson 3.8 – The Python Standard Library and pip ⇒