Introduction
In this article you’ll see in some detail one of the most widely used Python modules, the sys module.
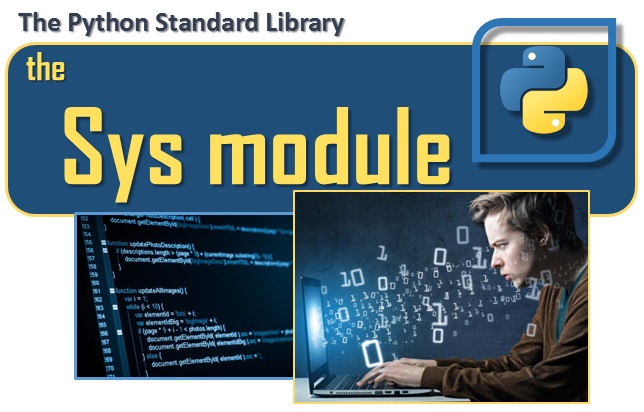
The sys module
This module is one of the basic packages included with the Python Standard Library. So once you installed Python (both the 2.7.x and 3.x.x version) just write
import sys
in order to import it into your code.
This module contains a number of functions and parameters that will be very useful whenever your program will have to interact with the operating system you’re working on. This will allow you to work on the file system, get various operating system information and perform other operations without having to worry about the operating system you’re working on.
For example it is very easy to obtain information about the version of the Python you’re working on.
sys.version

If you want to use these values for future controls in your program, you should use
sys.version_info
it returns the separate values for different attributes, so you can use them as individual control variables during the program.

Arguments for scripts at the command line
One of the main uses of this module is for scripts. In fact, often when you launch the execution of a Python program from the command line, you prefer to pass arguments, or options to the script, instead of modifying each time the values of variables in your code. Also creating scripts in this way makes them responsive to other shell commands launched via the command line.
The arguments passed to the command line will be enclosed within sys.argv in the form of a list. The first element of this list is the name of the script itself. The other elements follow the input orders for the command line.
Write the following code and save it as myscript.py.
#!/usr/bin/python import sys print "Script name: %s" % sys.argv[0] for i in range(len(sys.argv)): if i > 0: print "Arg%d: %s"% (i, sys.argv[i])
By running this script and passing a series of arguments you get:

Change the output behavior of the Python shell
One of the peculiar aspects of the programming in Python is the possibility of working in interactive mode. Activating a shell of Python is possible to introduce a line of code at a time and wait for the result and then enter the next. Every time you enter a command, the shell shows a significant output of the requested result.
Open a Python session and enter a command, such as assigning a value to a variable. You’ll get

This is the default behavior of a Python session, though sometimes you may need a different behavior. To change this behavior you can use sys.displayhook. You must pass a function that changes this default behavior.
import sys def new_display(x): print "out: ", x sys.displayhook = my_display

Standard Data Streams
If you already have some familiarity with operating systems such as Linux or Unix, certainly you know what they are. In fact, the standard data stream (also known as pipes) are three: one for the input values (stdin), one for the output messages (stdout) and one for the error messages (stderr).
With Python, precisely due to the sys module, you can directly access these data stream using sys.stdin, sys.stdout and sys.stderr.
sys.stdout.write("This is the output stream\n")
This command sends a string to the data stream output

As for the input, for example, you can pass a string in the data input stream that you will later request to the following command
x = sys.stdin.readline()[:-1]
Enter a string that will be stored in the variable x, with [-1] removes the newline character at the end of the string

If you want to add an error message to be inserted into the data stream error:
sys.stderr.write("This is the error message\n")

I/O Redirections
You’ve just seen the Standard Data Stream and how you can use them through the sys module. Another case related to them are the I / O redirections. This technique is used for those who work on Linux or Unix shell.
In fact, the standard data stream can be redirected to a file to make a read or a write on it. Even stderr often is redirected to a file to create a log file on which you can later check for error messages.
For example, creates a redirect.py file and insert the following code:
import sys sys.stdout.write("This is the output message\n") sys.stderr.write("This is the error message\n")
Now if you execute the program with the redirections of two log files
python redirect.py > output.log 2> error.log
You will find inside the two files corresponding messages.
Well all this is possible to do directly with Python within the program itself.
Create a redirect.py file and enter the following code.
import sys save_stdout = sys.stdout save_stderr = sys.stderr file1 = open("output.log","w") file2 = open("error.log","w") sys.stdout = file1 sys.stderr = file2 print("This is the output message\n") sys.stderr.write("This is the error message\n") sys.stdout = save_stdout sys.stderr = save_stderr file1.close() file2.close()
Some interesting parameters
Inside the sys module there are some attributes that let you meet some interesting parameters that may sometimes be useful.
For example
sys.executable
This attribute returns the path of the interpreter of Python on which you are running the program

sys.maxint
This attribute returns the largest positive integer supported by Python. (To get the biggest negative integer value using the stratagem “- maxint – 1”)

sys.modules
This dictionary contains all the modules that have been loaded by the interpreter of Python.
If you want access to a particular module you can write

sys.path
This attribute is a list of all the directories where Python searches for modules.

sys.platform
This attribute returns the name of the platform (operating system) on which Python is working

sys.getrecursionlimit()
sys.setrecursionlimit(limit)
These two functions are useful to see and set the limit of recursion possible accepted by the Python interpreter. This value is useful to prevent infinite recursion which would cause it to power down.

Conclusions
In this article you’ve seen an overview of the essential features and tools provided by this important module belonging to the Python Standard Library. I hope you found it useful and that this information can improve your approach to the world of programming in Python.
[:]