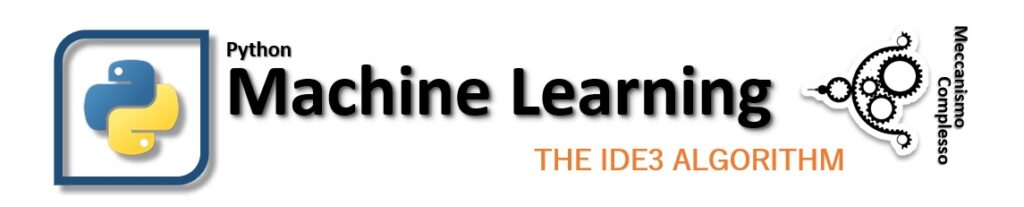
The IDE3 algorithm
The IDE3 (Iterative Dichotomiser 3) algorithm is a predecessor of the C4.5 algorithm and represents one of the first algorithms for building decision trees. Even though C4.5 and its successors have become more popular, IDE3 is still interesting because it helped lay the foundation for decision trees and machine learning. Below, I will explain how IDE3 works and how to use it in Python.
Operation of the IDE3 Algorithm:
IDE3 is a supervised learning algorithm used for building decision trees. Here’s how it works:
- Predictor Variable Selection: The IDE3 algorithm begins by selecting the predictor variable (feature) that provides the best split of the training data based on the target variable (class). Variable selection is based on entropy measurement or other impurity criteria.
- Data Splitting: Once the predictor variable is selected, IDE3 splits the training data based on the values of the predictor variable. Branches of the tree are created for each unique value of the variable.
- Recursion: The process of selecting the predictor variable and splitting the data is repeated recursively for each newly created branch. The algorithm continues to build the tree until a stopping criterion is met, such as a maximum depth reached or sufficient purity of leaf nodes.
- Creation of Leaf Nodes: When a node is reached where all examples belong to the same class (for classification problems) or the change in the target value is below a certain threshold (for regression problems), a node is created leaf representing the prediction.
Using IDE3 in Python:
While scikit-learn does not offer a direct implementation of IDE3, you can use the DecisionTreeClassifier algorithm to create entropy-based decision trees. Here is an example of how to do it:
from sklearn.tree import DecisionTreeClassifier from sklearn.datasets import load_iris from sklearn.model_selection import train_test_split from sklearn.metrics import accuracy_score #
Load the Iris datasetiris = load_iris() X = iris.data y = iris.target #
Divide the dataset into training and test setsX_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42) #
Create a decision tree model based on IDE3 (entropy)ide3_classifier = DecisionTreeClassifier(criterion="entropy", random_state=42) #
Train the model on the training dataide3_classifier.fit(X_train, y_train) #
Make predictions on test datay_pred = ide3_classifier.predict(X_test) #
Calculate the accuracy of the modelaccuracy = accuracy_score(y_test, y_pred) print("Accuratezza del modello:", accuracy)
In this example, we used the “entropy” criterion when we created the decision tree model to achieve similar behavior to IDE3. We then trained the model, made predictions, and calculated the accuracy.
Please note that IDE3 is primarily of historical and educational interest, as more modern implementations such as C4.5, CART, Random Forest, and Gradient Boosting Trees have become more popular and advanced.
A bit of history
The IDE3 (Iterative Dichotomiser 3) algorithm is one of the first algorithms for building decision trees and was developed by Ross Quinlan in the early 1980s. It is considered a predecessor of C4.5, one of the best-known and most influential decision tree algorithms.
Here is a brief summary of the history of IDE3:
- Initial Development: IDE3 was developed by Ross Quinlan during the early 1980s at the University of Sydney in Australia. The goal of IDE3 was to create a machine learning algorithm that could build decision trees for data classification.
- Key Idea: The key idea of IDE3 was to use the concept of entropy, derived from Claude Shannon’s information theory, to measure the impurity of a data set. IDE3 attempted to select the predictor variable that maximized entropy reduction, i.e. the variable that produced the best split of the data.
- Entropy and Recursive Splitting: IDE3 used entropy to calculate the information gain gained from splitting data based on a predictor variable. The algorithm then proceeded to create new branches in the tree for each unique value of the selected variable. This process ran recursively until a stopping criterion was met, such as leaf node purity.
- Contribution to Machine Learning: IDE3 was one of the pioneering algorithms in machine learning and laid the foundation for the development of subsequent algorithms such as C4.5. He demonstrated that it was possible to build decision trees automatically based on mathematical and statistical criteria rather than human rules.
- Evolution to C4.5: IDE3 was later followed by C4.5, which was developed by Quinlan as a significant improvement. C4.5 introduced improvements such as continuous variable handling and ratio-based variable selection, improving the flexibility and performance of decision trees.
- Educational Use: Although IDE3 is no longer used in real-world applications, it is still used for educational purposes to teach the basic concepts of decision trees and machine learning.
In summary, IDE3 was an important step in the history of machine learning, demonstrating the feasibility of building decision trees automatically. Its successor, C4.5, further developed these ideas and had an imp