Introduction
So far most of us have always believed that Raspberry Pi and Arduino as two competing platforms. Not by chance among various geeks and maker is not uncommon to show a bit of competition for Arduino fans against the Raspberry Pi fans. Well today I will slightly change things, and show you how you can take advantage of the best of everything. If you own both Arduino and Raspberry boards, this article will show you how to best and synergistically make use of these two boards.
Connecting Arduino to Raspberry
There are several ways to connect these two boards to each other, but the easiest way is just to use a USB connector.
If you want to see if the connection has been successful and Raspberry properly recognizes Arduino, from Raspbian open a terminal session and enter the following command to check if Arduino is in the list of devices connected via USB.
$ lsusb
Installating Arduino IDE in Raspbian
Always from the terminal enter the following commands
$ sudo apt-get update $ sudo apt-get install arduino
This will install an older version of the Arduino IDE, ie 1.0.5.
Otherwise you can use the Arduino IDE from any other PC to write programs and upload them to the Arduino board. Then you will simply connect the Arduino Raspberry Pi later
The overall scheme Raspberry Pi – Arduino
Now let’s look a bit at the big picture. Raspberry Pi is a very low-cost computers, not high performance, of course, but it still has its own Linux system, and performs all the necessary operations. This is all what you need. Maybe I would add the Wireless Dongle (of requirement;)).
Arduino is an electronic board that allows the control of sensors and gathering of their data. Also it is able to control actuators (motors) and switches. Ok, ok, 🙂 Raspberry also has its GPIO, but there are plenty of Arduino models suitable for every type of use and their cost is really low.
Arduino is an electronic board that allows the management of sensors and gathering of the their data. Also it is able to also control the actuators (motors) and switches. Ok, ok, 🙂 Raspberry also has its GPIO, but there are plenty of Arduino models suitable for every type of use and their cost is really low.
For example there is the Arduino Pro Mini which has dimensions of a few centimeters (like a thumb) and thus it can be easily used as a sensor controller.
Other Arduino models are flexible and can be connected to fabrics, soft surfaces, such as the LilyPad Arduino.
So in the scheme you’ll see many Arduino used as a controller for sensors and actuators, all connected to a single Raspberry Pi board. It can be regarded as a local station for management and data acquisition. Each Raspberry Pi will represent a local station of sensors and actuators that will transmit data via wireless to a central computer that could very well be your laptop.
In the figure below the diagram showing.
The role of Python in managing Arduino Data
Using Raspbian, you will use Python as the programming language in order to have a control to higher-level data coming and sent to the Arduino board. In fact, Python is a language that is well suited to the management of data both from the point of view of calculation that display. Python is In addition a language that allows you to execute commands both within programs in the form of code, or line by line from the terminal. These two approaches make Python the right tool for this kind of operation.
There are libraries such as Pandas for data analysis and Matplotlib for their visualization, which make Python an advanced tool for managing data.
For those who are beginners or wish to learn the analysis of the data subject, or even how to program in Python to generate eye-catching graphics, I highly recommend this book:
Python Data Analytics (Apress – 2015) – Fabio Nelli
This book starts from the Python language basics and then move on to an overview of the most Python libraries for data management: Numpy to have an efficient data structure underlying, Pandas that allows you to manage and manipulate data in a simple, efficient way, scikit-learn which uses the machine learning tools to make predictions and classifications on the collected data. Matplotlib that allows you to obtain 2D and 3D graphics for displaying data. Finally topics such as Data Mining and some classical approaches of the data analysis are discussed. The book is full of examples where the code is explained step by step.
Programming Arduino
Now for example, you will program an Arduino board (in my case Arduino UNO) to output data every 5 seconds. This data will be sent via serial and will then be collected and managed by Raspberry Pi.
Start the Arduino IDE that you just installed on Raspberry. Open a terminal session and enter the following command:
$ arduino
But before you start writing code, you’ll need to install the TIME library in order to include in your code the time.h header file. In fact this library, although very important, is not included in the standard libraries. To download it click here. Once you download the file time.zip, go on the IDE and import it with Sketch > Import Library > Add Library.
Then enter the following code:
Compile code and sent the program to Arduino that begins to run it iteratively.
From this moment Arduino repeatedly sends a signal every 5 seconds on the serial port, thus simulating the continuous detection by a sensor.
Programming Python with Raspberry Pi (Receiving data from Arduino)
Now open a terminal session. You will work with Python 2.7. pySerial is the library needed to communicate with Arduino and manage the serial data sent from it. Fortunately, this library is already included in the base distribution and thus you will not need to install it.
Edit the code below with nano, or any other text editor, and save it as arduino01.py.
import serial arduinoSerialData = serial.Serial('/dev/ttyACM0',9600) while 1: if(arduinoSerialData.inWaiting()>0): myData = arduinoSerialData.readline() print myData
Execute the code, with the command:
$ python arduino01.py
Now the signal sent from the Arduino will be received by Raspberry through the serial port (USB) ttyACM0. Warning, if you have more Arduino connected, the Arduino sending could have another assigned serial port (please check).
A line of text will appear on the terminal every 5 seconds that shows the message sent from Arduino.
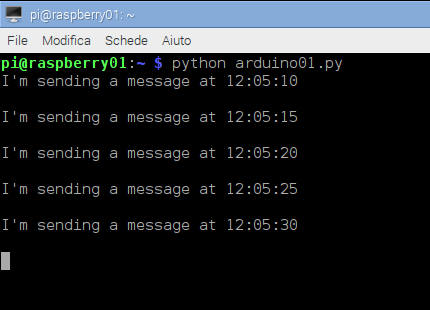
Programming Python on Raspberry (Sending commands to Arduino)
In the previous example you received from Raspberry signals from an Arduino board simulating the reading of values from a sensor. Now in this example, you will see the opposite case. You saw that in the Arduino-Raspberry diagram shown in this article, the Raspberry boards perform the task of controlling one or more Arduino, to which not only sensors are connected, but also actuators or switches.
Then Raspberry, by means Python, will be able not only to receive data from the Arduino via the serial port, but also to send commands so they can be performed by Arduino. Commands that will be used to stop reading, start an engine, close a switch, etc.
In this example, you will write a code that puts the Arduino board waiting, ready to receive commands from serial line. The commands will be numbers between 0 and 9, which correspond to the frequency with which the LED 13 (the one built into the Arduino board) will blink.
Open the Arduino IDE and write the following code.
Once you have finished, compile and upload it to the Arduino board, which will begin to execute the program. Arduino will listen ready to receive a command from Raspberry.
Before you saw how you could manage communication with Arduino through a program in Python. Now in this example, you’ll see the interactive approach through the terminal. In fact, the Python commands can be launched line by line.
First, you have to lanch Python as interpreter.
$ python
and then you can enter a command at once and see what is happening (useful, is it true?).
>>> import serial >>> arduinoSerialData = serial.Serial('/dev/ttyACM0',9600) >>> arduinoSerialData.write('5')
After this last command you will see the LED blinking on the Arduino board with a normal frequency.
>>> arduinoSerialData.write('3')
Now you will see the LED blinking more repeatedly.
Conclusions
In this article you’ve seen how it is possible to work synergistically with the Raspberry Pi and Arduino boards. In a future article I will discuss the case where two Arduino will be managed simultaneously from the same Raspberry board. These two Arduino will produce a series of data that will be collected from Raspberry, processed through the Python libraries such as Pandas and finally displayed in graphs through Matplotlib.